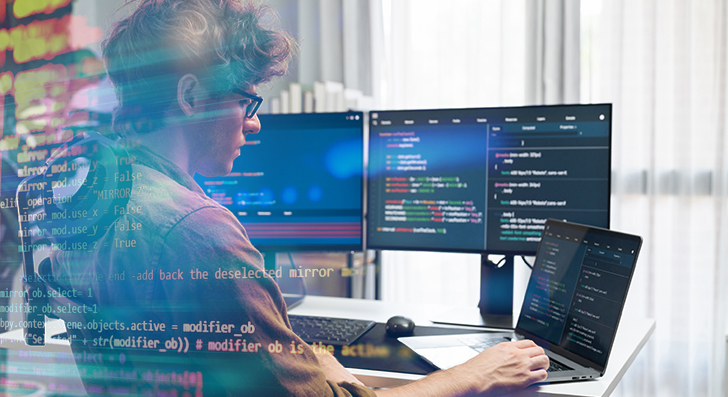
Scalability implies your software can cope with progress—much more users, additional knowledge, and a lot more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety afterwards. Below’s a clear and sensible guideline to assist you to commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be component of your respective program from the start. Several purposes fail if they expand speedy since the first style can’t cope with the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Start by planning your architecture to be flexible. Prevent monolithic codebases where almost everything is tightly related. Rather, use modular layout or microservices. These patterns break your app into scaled-down, unbiased components. Every single module or company can scale on its own without having impacting The complete system.
Also, take into consideration your database from working day a person. Will it need to deal with 1,000,000 end users or simply just 100? Choose the correct sort—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t generate code that only works under current circumstances. Consider what would take place When your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use structure styles that aid scaling, like information queues or celebration-pushed programs. These aid your app deal with much more requests with out getting overloaded.
When you Establish with scalability in your mind, you're not just getting ready for success—you might be lessening long run complications. A effectively-planned system is less complicated to keep up, adapt, and expand. It’s much better to arrange early than to rebuild later on.
Use the correct Database
Deciding on the appropriate database is a vital Component of constructing scalable applications. Not all databases are crafted the exact same, and using the wrong you can slow you down or simply lead to failures as your app grows.
Get started by comprehension your information. Can it be very structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your facts is more versatile—like user action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling huge volumes of unstructured or semi-structured data and may scale horizontally extra effortlessly.
Also, look at your read and compose styles. Are you currently undertaking a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Explore databases which will tackle higher publish throughput, or maybe event-primarily based info storage devices like Apache Kafka (for non permanent data streams).
It’s also intelligent to Consider forward. You might not will need advanced scaling attributes now, but selecting a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access patterns. And usually check database efficiency as you develop.
In brief, the correct database depends upon your app’s structure, velocity requires, And exactly how you be expecting it to improve. Acquire time to choose properly—it’ll conserve lots of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, uncomplicated code. Keep away from repeating logic and remove anything unwanted. Don’t select the most complex Alternative if an easy one works. Maintain your functions shorter, targeted, and easy to check. Use profiling tools to uncover bottlenecks—areas where your code can take also long to run or uses an excessive amount memory.
Subsequent, evaluate your database queries. These normally slow matters down over the code alone. Make certain Just about every query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find particular fields. Use indexes to hurry up lookups. And avoid carrying out too many joins, Specially throughout big tables.
In case you see the identical facts being requested over and over, use caching. Retail store the outcomes briefly working with applications like Redis or Memcached so that you don’t should repeat expensive operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and tends to make your app far more successful.
Make sure to exam with large datasets. Code and queries that perform wonderful with one hundred data could crash every time they have to handle 1 million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when required. These measures aid your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more visitors. If every thing goes via 1 server, it'll rapidly become a bottleneck. That’s where by load balancing and caching are available. Both of these equipment aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming targeted traffic across several servers. Rather than 1 server doing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This suggests no solitary server will get overloaded. If one server goes down, the load balancer can mail traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused swiftly. When customers ask for the identical information yet again—like a product web site or possibly a profile—you don’t have to fetch it with the database when. It is possible to serve it through the cache.
There are two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and tends to make your application more productive.
Use caching for things which don’t alter generally. And always ensure that your cache is updated when knowledge does improve.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app take care of extra customers, keep speedy, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you'll need them. You don’t must get hardware or guess foreseeable future ability. When targeted traffic boosts, you may insert additional methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and safety resources. You are able to concentrate on building your application in place of controlling infrastructure.
Containers are Yet another important tool. A container packages your application and almost everything it should run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Once your application utilizes various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it quickly.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, working with cloud and container resources usually means you'll be able to scale speedy, deploy very easily, and Get better swiftly when complications occur. If you prefer your app to increase without limitations, get started utilizing these instruments early. They save time, minimize possibility, and assist you to keep centered on building, not repairing.
Watch Everything
Should you don’t watch your software, you won’t know when items go Mistaken. Checking helps you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a vital A part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Room, and response time. These inform you how your servers and expert services are accomplishing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your app way too. Control just how long it requires for end users to load web pages, how frequently problems come about, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly here can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This assists you fix issues speedy, generally ahead of consumers even discover.
Checking is likewise valuable once you make modifications. If you deploy a different attribute and see a spike in faults or slowdowns, you may roll it back before it will cause actual damage.
As your application grows, site visitors and data raise. Without having monitoring, you’ll miss out on indications of difficulty right until it’s way too late. But with the proper applications in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant firms. Even compact apps will need a powerful Basis. By designing meticulously, optimizing wisely, and using the suitable resources, you may Develop apps that mature smoothly with no breaking stressed. Start modest, Imagine large, and Create good.